Prelude
Today I want to deep dive into how you can leverage AWS Amplify, a tool that provides a comprehensive set of tools and services that streamline the process of developing and deploying web applications on AWS infrastructure: we will explore how to build a web application served by AWS API Gateway and Lambda functions. We will walk through the steps of initializing an Amplify project, adding Lambda functions, linking them to an API, and implementing authentication mechanisms.
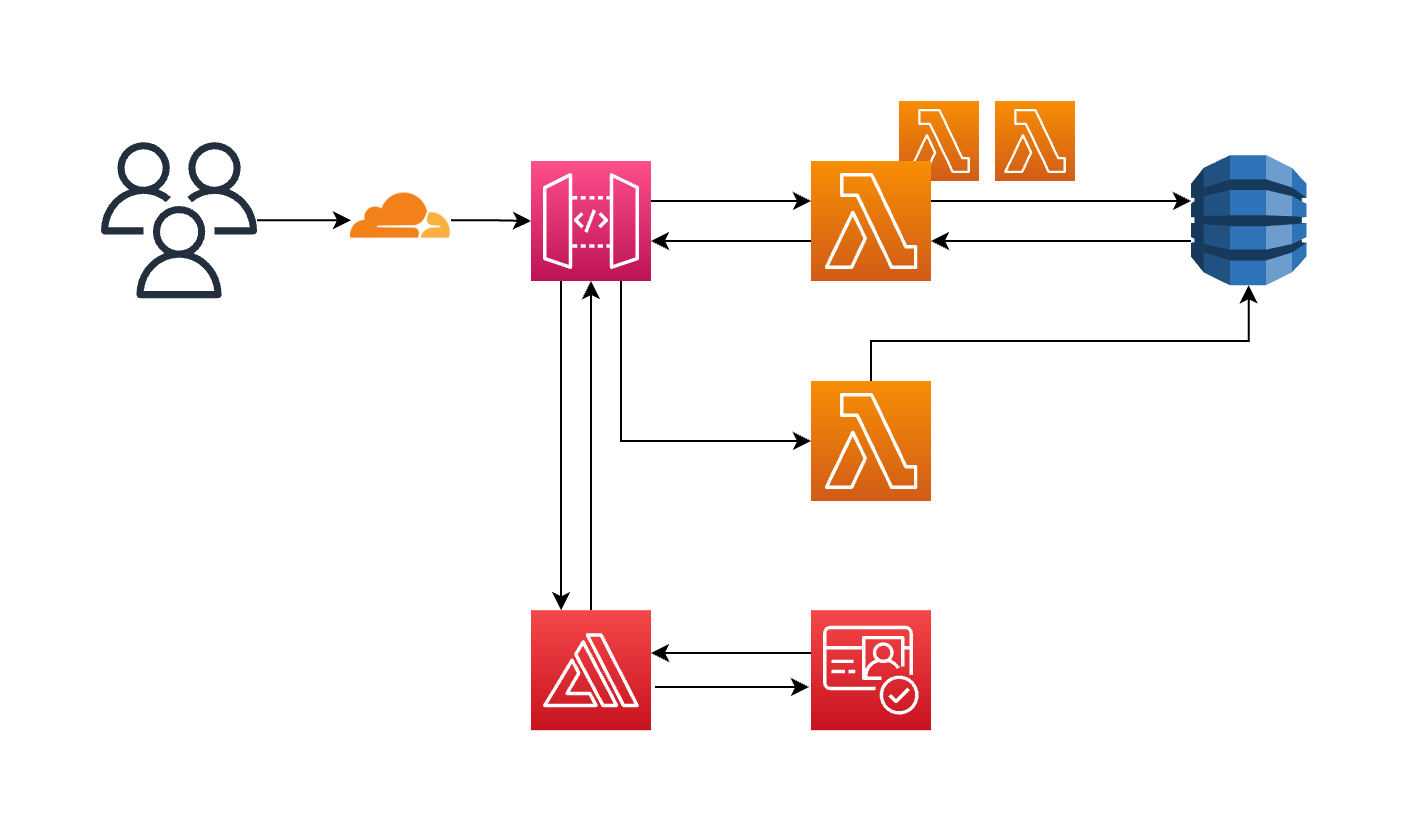
Introduction to AWS Amplify
AWS Amplify is a set of tools and services that enable developers to build full-stack web applications quickly and easily. It provides a wide range of features, including authentication, data storage, API integration, and hosting. With Amplify, developers can focus on building innovative features for their applications while offloading infrastructure management and scalability concerns to AWS. You can find more info here
Setting up the Project with AWS Amplify
Let’s start by initializing a new Amplify project for our web application. Ensure that you have the AWS Amplify CLI installed on your local machine. If not, you can install it using npm:
npm install -g @aws-amplify/cli
Once installed, navigate to your project directory and run the following command to initialize a new Amplify project:
amplify init
Follow the prompts to configure your Amplify project. You will be asked to provide a name for your project, select the default editor, and choose the AWS profile you want to use.
Adding Lambda Functions
Now that we have our Amplify project set up, let’s add some Lambda functions to handle the business logic of our application. Lambda functions are serverless compute services provided by AWS that allow you to run code without provisioning or managing servers.
To add a new Lambda function to our Amplify project, run the following command:
amplify add function
Follow the prompts to configure your Lambda function. You will be asked to provide a name for your function, choose the runtime environment (e.g., Node.js), and specify the function handler and other settings.
Repeat this process for each Lambda function you want to add to your project.
Linking Lambda Functions to API Gateway
Next, let’s link our Lambda functions to an API Gateway to expose them as RESTful endpoints. API Gateway is a fully managed service provided by AWS that makes it easy to create, publish, maintain, monitor, and secure APIs at any scale.
To link a Lambda function to API Gateway, run the following command:
amplify add api
Follow the prompts to configure your API. You will be asked to provide a name for your API, choose the authorization type (e.g., API key, AWS IAM), and specify the paths and Lambda functions associated with each endpoint.
? Please select from one of the below mentioned services:
`REST`
? Provide a friendly name for your resource to be used as a label for this category in the project:
`todoApi`
? Provide a path (e.g., /book/{isbn}):
`/todo`
? Choose a Lambda source
`Create a new Lambda function`
? Provide the AWS Lambda function name:
`todoFunction`
? Choose the function runtime that you want to use:
`NodeJS`
? Choose the function template that you want to use:
`Serverless ExpressJS function (Integration with API Gateway)`
? Do you want to access other resources created in this project from your Lambda function?
`No`
? Do you want to invoke this function on a recurring schedule?
`No`
? Do you want to edit the local lambda function now?
`No`
? Restrict API access
`No`
? Do you want to add another path?
`No`
Implementing Authentication with AWS Amplify
Now that we have our API set up, let’s implement authentication for our web application using AWS Amplify. Amplify provides built-in authentication mechanisms that make it easy to add user authentication to your applications.
To add authentication to your Amplify project, run the following command:
amplify add auth
Follow the prompts to configure authentication for your project. You will be asked to choose the authentication type (e.g., Amazon Cognito user pools, Amazon Cognito identity pools) and specify the settings for user sign-up, sign-in, and multi-factor authentication.
Once authentication is set up, you can use the Amplify SDK to integrate authentication into your web application. For example, you can use the Auth
module to handle user authentication, sign-up, sign-in, and sign-out operations.
Add amplify auth inside your webapp
To add amplify auth, you can use Amplify official package by loading the configuration of amplify inside your app entrypoint (specifically, App.js
, index.js
, _app.js
, or main.js
). The file of the configuration is called amplifyconfiguration.json
or aws-exports.js
depending on your amplify version.
import { Amplify } from 'aws-amplify';
import config from './amplifyconfiguration.json';
Amplify.configure(config);
Inside your component you can do something like this:
import { Auth } from 'aws-amplify';
export default {
... <the rest of your vue component for a login page> ...
methods: {
async signIn() {
this.loading = true;
await Auth.signIn(this.email, this.password).then((user) => {
this.store.commit('signIn', user);
console.log("signIn - fine", user);
this.loading = false;
syncSubscriptionPlan(this.store); // we are gonna explain this in the future ;)
this.$router.push({ path: '/' });
}).catch((error) => {
this.errorMessage = error;
this.loginAlert = true;
this.loading = false;
console.log("signIn - error", error);
return null;
});
}
},
... <the rest of your vue component for a login page> ...
}
Deploying the Web Application
With our Lambda functions, API Gateway, and authentication set up, we are ready to deploy our web application. Run the following command to deploy your Amplify project:
amplify push
Amplify will provision the necessary resources on AWS, including Lambda functions, API Gateway endpoints, authentication services, and any other configured resources.
Once the deployment is complete, you can access your web application using the provided URL. You can also continue to develop and iterate on your application by making changes to your code and running the amplify push command to deploy the updates.
Pro and Cons
AWS Amplify comes with various pros and cons that developers should consider when choosing it for their projects:
simplified development: AWS Amplify simplifies the development process by providing a unified platform for managing backend resources, authentication, and frontend components. Developers can focus on building features rather than managing infrastructure.
rapid prototyping: With Amplify’s intuitive CLI and pre-built UI components, developers can quickly prototype and iterate on their applications. This accelerates the development cycle and allows for faster time-to-market.
built-in authentication: Amplify offers built-in authentication mechanisms, including Amazon Cognito user pools and identity pools, making it easy to add user authentication and authorization to applications. This helps developers ensure the security of their applications without writing complex authentication logic.
seamless integration: Amplify seamlessly integrates with other AWS services, such as API Gateway, Lambda functions, and DynamoDB, allowing developers to leverage the full power of the AWS ecosystem in their applications.
On the other hand, what I found a cons are:
limited customization: While Amplify simplifies the development process, it may limit customization options for developers who require more control over their infrastructure or want to use non-AWS services.
learning curve: Despite its ease of use, Amplify has a learning curve, especially for developers who are new to AWS or serverless architecture concepts. It may take time for developers to become proficient in using Amplify effectively.
complexity for large projects: While Amplify is well-suited for small to medium-sized projects, it may become complex to manage for large-scale applications with intricate requirements. Developers may need to supplement Amplify with custom solutions or additional services to meet their project’s needs.
For the first poin however, I just discovered that…
Amplify Gen 2
Recently AWS released AWS Amplify Gen 2. As stated in the doc, AWS Amplify (Gen 2) introduces a TypeScript-based, code-first developer experience (DX) for defining backends. The Gen-2 DX offers a unified Amplify developer experience with the same hosting, backend, and UI-building capabilities previously available in CLI and Studio, but with a code-first approach. Amplify empowers frontend developers to deploy cloud infrastructure by simply expressing their app’s data model, business logic, authentication, and authorization rules completely in TypeScript. Amplify automatically configures the correct cloud resources and removes the requirement to stitch together underlying AWS services.
Maybe I will try it and let you know if it works better than preview generation!
Conclusion
In this article, we explored how to leverage AWS Amplify to build a web application served by AWS API Gateway and Lambda functions. We walked through the process of initializing an Amplify project, adding Lambda functions, linking them to an API, and implementing authentication mechanisms. I didn’t create a DynamoDB table but you can do it as a custom resource without problems… we are gonna see how to do it properly in a next article!
By using Amplify, developers can rapidly develop and deploy scalable and secure web applications on AWS infrastructure. With its built-in features and services, Amplify simplifies the development process and allows developers to focus on building innovative features for their applications.
For more information on AWS Amplify and its capabilities, refer to the official documentation and resources provided by AWS. Happy coding and… stay tuned for the next steps!
If you lost the first article of the serie, have a look at building an e-learning platform on AWS. Otherwise, move to the next article about how to produce HLS content ready for streaming with AWS Media Convert